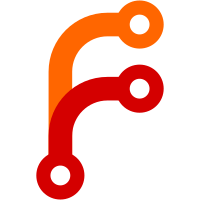
Bots are not part of what we distribute, so put them in the repo root. We also updated some of the bots to use relative path names. (imported from commit 0471d863450712fd0cdb651f39f32e9041df52ba)
51 lines
1.7 KiB
Python
Executable file
51 lines
1.7 KiB
Python
Executable file
#!/usr/bin/env python
|
|
import sys
|
|
import optparse
|
|
from os import path
|
|
|
|
sys.path.append(path.join(path.dirname(__file__), '../api'))
|
|
import humbug
|
|
|
|
# Nagios passes the notification details as command line options.
|
|
# In Nagios, "output" means "first line of output", and "long
|
|
# output" means "other lines of output".
|
|
parser = optparse.OptionParser()
|
|
parser.add_option('--output', default='')
|
|
parser.add_option('--long-output', default='')
|
|
for opt in ('type', 'host', 'service', 'state'):
|
|
parser.add_option('--' + opt)
|
|
(opts, args) = parser.parse_args()
|
|
|
|
msg = dict(type='stream', to='nagios')
|
|
|
|
# Set a subject based on the host or service in question. This enables
|
|
# threaded discussion of multiple concurrent issues, and provides useful
|
|
# context when narrowed.
|
|
#
|
|
# We send PROBLEM and RECOVERY messages to the same subject.
|
|
if opts.service is None:
|
|
# Host notification
|
|
thing = 'host'
|
|
msg['subject'] = 'host %s' % (opts.host,)
|
|
else:
|
|
# Service notification
|
|
thing = 'service'
|
|
msg['subject'] = 'service %s on %s' % (opts.service, opts.host)
|
|
|
|
# e.g. **PROBLEM**: service is CRITICAL
|
|
msg['content'] = '**%s**: %s is %s' % (opts.type, thing, opts.state)
|
|
|
|
# The "long output" can contain newlines represented by "\n" escape sequences.
|
|
# The Nagios mail command uses /usr/bin/printf "%b" to expand these.
|
|
# We will be more conservative and handle just this one escape sequence.
|
|
output = (opts.output + '\n' + opts.long_output.replace(r'\n', '\n')).strip()
|
|
if output:
|
|
# Put any command output in a code block.
|
|
msg['content'] += ('\n\n~~~~\n' + output + "\n~~~~\n")
|
|
|
|
client = humbug.Client(
|
|
email = 'humbug+nagios@humbughq.com',
|
|
api_key = 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx',
|
|
site = 'https://staging.humbughq.com')
|
|
client.send_message(msg)
|