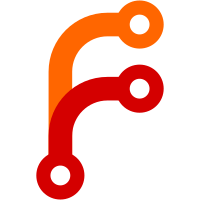
This test uses unittest and mock library. It mocks 'BotHandlerApi' class. This test works independent of the rest of the code outside contrib_bots folder. Merged with a few changes by tabbott to fix lint issues; we'll need to do further work on this framework, but since it's not hooked up to anything, it's reasonable to merge early so others can collaborate on improving it.
44 lines
1.5 KiB
Python
44 lines
1.5 KiB
Python
#!/usr/bin/env python
|
|
|
|
from __future__ import absolute_import
|
|
from __future__ import print_function
|
|
|
|
import os
|
|
import sys
|
|
import unittest
|
|
|
|
from mock import MagicMock, patch
|
|
from unittest import TestCase
|
|
|
|
from run import get_lib_module
|
|
from bot_lib import StateHandler
|
|
from contrib_bots import bot_lib
|
|
from six.moves import zip
|
|
|
|
class BotTestCase(TestCase):
|
|
|
|
def mock_test(self, messages, message_handler, bot_response):
|
|
# type: (List[Dict[str, str]], Function) -> None
|
|
# Mocking BotHandlerApi
|
|
with patch('contrib_bots.bot_lib.BotHandlerApi') as MockClass:
|
|
instance = MockClass.return_value
|
|
|
|
for (message, response) in zip(messages, bot_response):
|
|
# Send message to the concerned bot
|
|
message_handler.handle_message(message, MockClass(), StateHandler())
|
|
|
|
# Check if BotHandlerApi is sending a reply message.
|
|
# This can later be modified to assert the contents of BotHandlerApi.send_message
|
|
instance.send_reply.assert_called_with(message, response)
|
|
|
|
def bot_to_run(self, bot_module):
|
|
# type: None -> Function
|
|
lib_module = get_lib_module(bot_module)
|
|
message_handler = lib_module.handler_class()
|
|
return message_handler
|
|
|
|
def bot_test(self, messages, bot_module, bot_response):
|
|
|
|
message_handler = self.bot_to_run(bot_module)
|
|
self.mock_test(messages=messages, message_handler=message_handler, bot_response=bot_response)
|