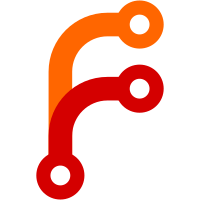
Personals are now just private messages between two people (which sometimes manifests as a private message with one recipient). The new message type on the send path is 'private'. Note that the receive path still has 'personal' and 'huddle' message types. (imported from commit 97a438ef5c0b3db4eb3e6db674ea38a081265dd3)
146 lines
4.6 KiB
Python
Executable file
146 lines
4.6 KiB
Python
Executable file
#!/usr/bin/python
|
|
import sys
|
|
import time
|
|
import optparse
|
|
import os
|
|
import random
|
|
|
|
parser = optparse.OptionParser()
|
|
parser.add_option('--verbose',
|
|
dest='verbose',
|
|
default=False,
|
|
action='store_true')
|
|
parser.add_option('--site',
|
|
dest='site',
|
|
default="https://humbughq.com",
|
|
action='store')
|
|
parser.add_option('--root-path',
|
|
dest='root_path',
|
|
default="/home/humbug",
|
|
action='store')
|
|
(options, args) = parser.parse_args()
|
|
|
|
sys.path[:0] = [os.path.join(options.root_path, "python-zephyr"),
|
|
os.path.join(options.root_path, "python-zephyr/build/lib.linux-x86_64-2.6/"),
|
|
options.root_path]
|
|
|
|
mit_user = 'tabbott/extra@ATHENA.MIT.EDU'
|
|
humbug_user = 'tabbott/extra@mit.edu'
|
|
|
|
zhkey1 = random.getrandbits(32)
|
|
hzkey1 = random.getrandbits(32)
|
|
zhkey2 = random.getrandbits(32)
|
|
hzkey2 = random.getrandbits(32)
|
|
|
|
sys.path.append(".")
|
|
sys.path.append(os.path.dirname(os.path.dirname(__file__)))
|
|
import api.common
|
|
humbug_client = api.common.HumbugAPI(email=humbug_user,
|
|
api_key="xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx",
|
|
verbose=True,
|
|
client="test: Humbug API",
|
|
site=options.site)
|
|
|
|
def print_zephyr(notice):
|
|
print notice.cls, notice.instance, notice.sender, notice.message.split('\0')[1]
|
|
|
|
def print_humbug(message):
|
|
if message['type'] == "stream":
|
|
print message["type"], message['display_recipient'], message['subject'], \
|
|
message['sender_email'], message['content']
|
|
else:
|
|
print message["type"], message['sender_email'], \
|
|
message['display_recipient'], message['content']
|
|
|
|
child_pid = os.fork()
|
|
if child_pid == 0:
|
|
# Run the humbug => zephyr mirror in the child
|
|
time.sleep(3)
|
|
humbug_client.send_message({
|
|
"type": "private",
|
|
"content": str(hzkey1),
|
|
"recipient": humbug_user,
|
|
});
|
|
time.sleep(0.2)
|
|
humbug_client.send_message({
|
|
"type": "stream",
|
|
"subject": "test",
|
|
"content": str(hzkey2),
|
|
"stream": "tabbott-nagios-test",
|
|
});
|
|
if options.verbose:
|
|
print "Sent Humbug messages!"
|
|
time.sleep(0.5)
|
|
|
|
import zephyr
|
|
zephyr.init()
|
|
zsig = "Timothy Good Abbott"
|
|
|
|
zeph = zephyr.ZNotice(sender=mit_user, auth=True, recipient=mit_user,
|
|
cls="message", instance="personal")
|
|
zeph.setmessage("%s\0%s" % (zsig, zhkey1))
|
|
zeph.send()
|
|
time.sleep(0.2)
|
|
|
|
zeph = zephyr.ZNotice(sender=mit_user, auth=True,
|
|
cls="tabbott-nagios-test", instance="test")
|
|
zeph.setmessage("%s\0%s" % (zsig, zhkey2))
|
|
zeph.send()
|
|
if options.verbose:
|
|
print "Sent Zephyr messages!"
|
|
|
|
|
|
else:
|
|
failed = False
|
|
import zephyr
|
|
zephyr.init()
|
|
subs = zephyr.Subscriptions()
|
|
subs.add(('message', 'personal', 'tabbott/extra@ATHENA.MIT.EDU'))
|
|
subs.add(('tabbott-nagios-test', '*', '*'))
|
|
|
|
max_message_id = humbug_client.get_profile()['max_message_id']
|
|
|
|
time.sleep(10)
|
|
if options.verbose:
|
|
print "Receiving messages!"
|
|
notices = []
|
|
while True:
|
|
notice = zephyr.receive(block=False)
|
|
if notice is None:
|
|
break
|
|
if notice.opcode != "":
|
|
continue
|
|
notices.append(notice)
|
|
if len(notices) != 4:
|
|
print "humbug=>zephyr: Got wrong number of messages back!"
|
|
failed = True
|
|
elif (set(notice.message.split('\0')[1] for notice in notices) !=
|
|
set([str(hzkey1), str(hzkey2), str(zhkey1), str(zhkey2)])):
|
|
print "humbug=>zephyr: Didn't get back right values!"
|
|
failed = True
|
|
if failed:
|
|
for notice in notices:
|
|
print_zephyr(notice)
|
|
|
|
messages = humbug_client.get_messages({'first': '0',
|
|
'last': str(max_message_id),
|
|
'server_generation': '0'})['messages']
|
|
if len(messages) != 4:
|
|
print "zephyr=>humbug: Didn't get exactly 4 messages!"
|
|
for message in messages:
|
|
print_humbug(message)
|
|
failed = True
|
|
elif (set(message["content"] for message in messages) !=
|
|
set([str(hzkey1), str(hzkey2), str(zhkey1), str(zhkey2)])):
|
|
print "zephyr=>humbug: Didn't get back right values!"
|
|
for message in messages:
|
|
print_humbug(message)
|
|
failed = True
|
|
|
|
if failed:
|
|
print "original keys:", zhkey1, zhkey2, hzkey1, hzkey2
|
|
sys.exit(1)
|
|
|
|
print "Success!"
|
|
sys.exit(0)
|